The provided code adds a secure custom passcode field to WordPress user profiles, allowing administrators to assign, save, and display secret passcodes while ensuring data protection through nonce verification, and input sanitization.
Example Picture
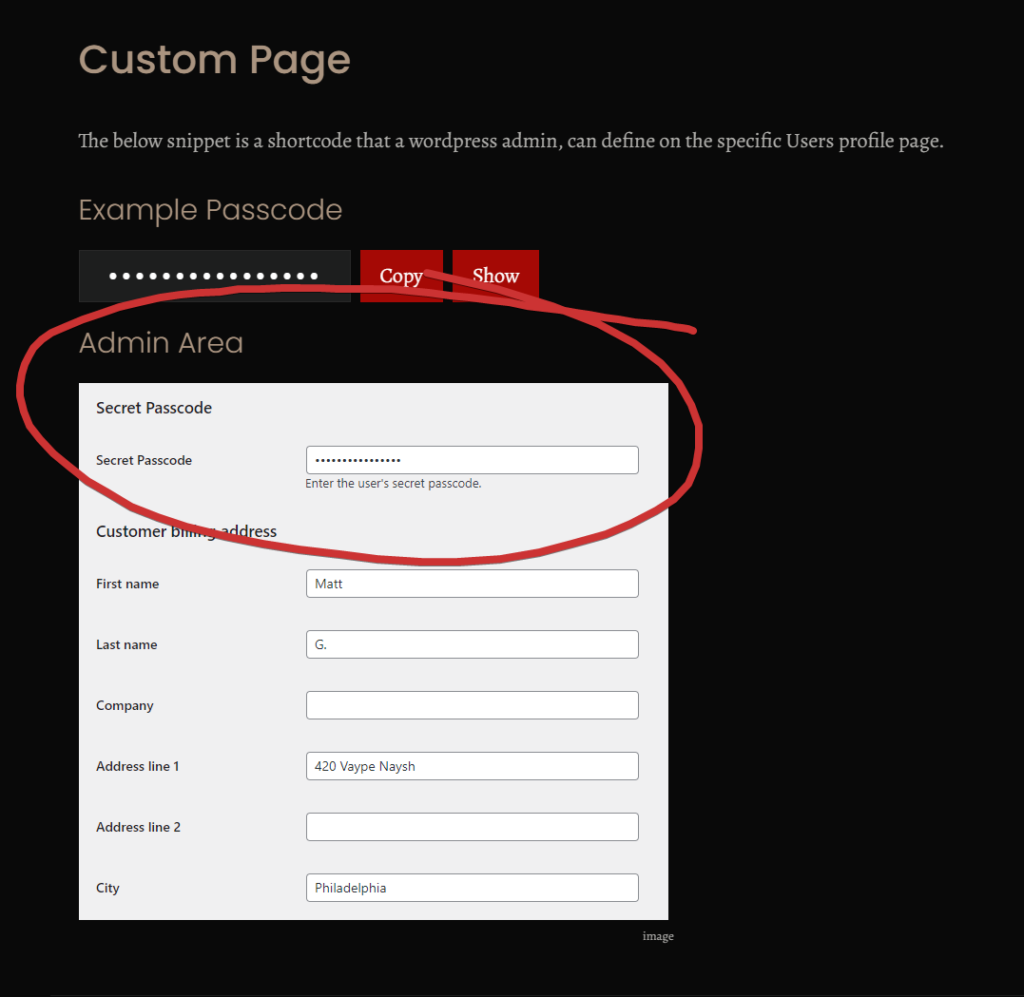
It includes functionality for users to reveal their passcodes and easily copy them to the clipboard, enhancing user experience without compromising security.
- Install & activate this plugin Code Snippets than copy / paste the snippet below:
- Create a page and paste the shortcode [ user_passcode ] on it.
- Visit user profile page as admin, and edit the field
- Logged in user visits page and can copy / paste the passphrase
// Add a custom passcode field to the user profile page in the admin
function add_custom_passcode_field( $user ) {
?>
<h3><?php _e('Secret Passcode', 'your-textdomain'); ?></h3>
<table class="form-table">
<tr>
<th><label for="secret_passcode"><?php _e('Secret Passcode'); ?></label></th>
<td>
<input type="password" name="secret_passcode" id="secret_passcode" value="<?php echo esc_attr( get_user_meta( $user->ID, 'secret_passcode', true ) ); ?>" class="regular-text" /><br />
<span class="description"><?php _e('Enter the user\'s secret passcode.'); ?></span>
<?php wp_nonce_field('save_passcode_nonce', 'passcode_nonce'); // Add nonce for security ?>
</td>
</tr>
</table>
<?php
}
add_action( 'show_user_profile', 'add_custom_passcode_field' );
add_action( 'edit_user_profile', 'add_custom_passcode_field' );
// Save the custom passcode field when the profile is updated
function save_custom_passcode_field( $user_id ) {
// Check if the user has permission to edit the profile
if ( !current_user_can( 'edit_user', $user_id ) ) {
return false;
}
// Verify the nonce
if ( !isset($_POST['passcode_nonce']) || !wp_verify_nonce($_POST['passcode_nonce'], 'save_passcode_nonce') ) {
return;
}
// Save or update the passcode in user meta
if ( isset( $_POST['secret_passcode'] ) ) {
// Sanitize and hash the passcode before saving (if applicable)
$hashed_passcode = sanitize_text_field( $_POST['secret_passcode'] ); // Hashing can be implemented as needed
update_user_meta( $user_id, 'secret_passcode', $hashed_passcode );
}
}
add_action( 'personal_options_update', 'save_custom_passcode_field' );
add_action( 'edit_user_profile_update', 'save_custom_passcode_field' );
// Automatically generate a passcode for new users on registration (optional)
function assign_passcode_on_registration( $user_id ) {
$passcode = wp_generate_password( 12, false ); // Generate a 12-character passcode
update_user_meta( $user_id, 'secret_passcode', sanitize_text_field( $passcode ) ); // Store the generated passcode
}
add_action( 'user_register', 'assign_passcode_on_registration' );
// Shortcode to display the user's secret passcode with password field
function display_user_passcode() {
// Check if the user is logged in
if ( is_user_logged_in() ) {
$user_id = get_current_user_id(); // Get the current user's ID
$passcode = get_user_meta( $user_id, 'secret_passcode', true ); // Retrieve the passcode
// Check if a passcode is set, otherwise show a fallback message
if ( $passcode ) {
return '
<div class="passcode-container">
<input type="password" id="passcode-input" class="passcode-input" value="' . esc_html( $passcode ) . '" readonly>
<button class="copy-passcode-btn" onclick="copyPasscode()">Copy</button>
<button class="reveal-passcode-btn" onclick="togglePasscode()">Show</button>
</div>';
} else {
return 'No passcode assigned to your account.';
}
} else {
return 'You need to log in to view your passcode.';
}
}
add_shortcode( 'user_passcode', 'display_user_passcode' );
// Enqueue necessary styles and JavaScript for the reveal and copy functionality
function enqueue_passcode_scripts() {
?>
<style>
/* Styling for passcode container, input field, and buttons */
.passcode-container {
display: flex;
align-items: center;
gap: 10px;
}
.passcode-input {
letter-spacing: 2px;
font-family: monospace;
color: #FFF !important;
text-align: center;
}
.reveal-passcode-btn, .copy-passcode-btn {
cursor: pointer;
}
.reveal-passcode-btn:hover, .copy-passcode-btn:hover {
/* Add hover effects for better UX */
background-color: #0073aa;
color: #fff;
}
</style>
<script>
// JavaScript function to toggle the passcode visibility
function togglePasscode() {
var passcodeInput = document.getElementById("passcode-input");
var revealBtn = document.querySelector(".reveal-passcode-btn");
// Toggle the type attribute between 'password' and 'text'
if (passcodeInput.type === "password") {
passcodeInput.type = "text";
revealBtn.textContent = "Hide"; // Change button text to 'Hide'
} else {
passcodeInput.type = "password";
revealBtn.textContent = "Show"; // Change button text to 'Show'
}
}
// JavaScript function to copy the passcode to clipboard
function copyPasscode() {
var passcodeInput = document.getElementById("passcode-input");
passcodeInput.select(); // Select the text
passcodeInput.setSelectionRange(0, 99999); // For mobile devices
// Copy the text inside the input field
document.execCommand("copy");
// Optionally provide user feedback
document.querySelector(".copy-passcode-btn").textContent = "Copied!";
setTimeout(() => {
document.querySelector(".copy-passcode-btn").textContent = "Copy";
}, 2000); // Reset button text after 2 seconds
}
</script>
<?php
}
add_action( 'wp_footer', 'enqueue_passcode_scripts' );
This could be good if you are offering a service to someone and want to share private info behind a login screen and don’t want to use email… maybe a password to email account, or a download link to a file that isn’t hosted on Dropbox or login credentials for a hosting account… TONS of ideas…